Exaud Blog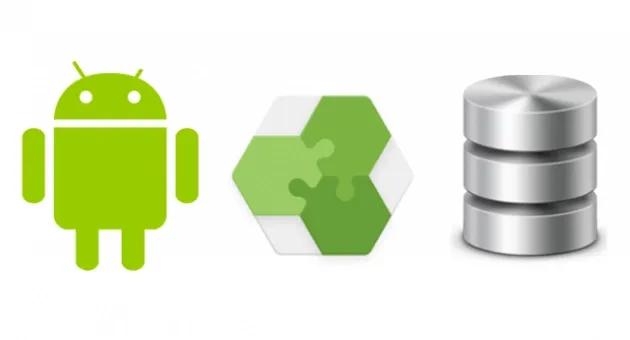
Blog
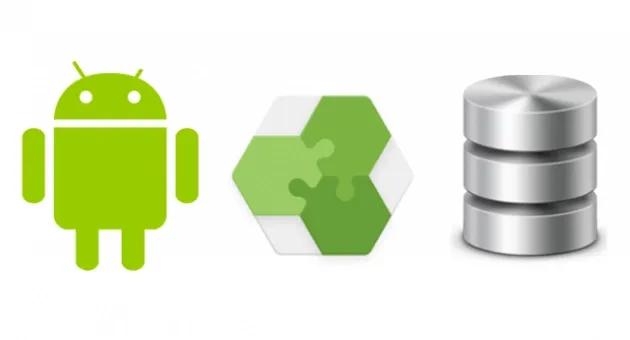
Building Database with Room Persistence Library
Room is a new way to create a database in your android apps. Learn how to reduce time and effort developing your app. Posted onby André LopesRoom is a new way to create a database in your android apps. Learn how to reduce time and effort developing your app.
Room Persistence Library is a library that adds an abstraction layer over SQLite which simplifies the access to the database, reduces the boilerplate and validates the SQL queries at runtime.
At the beginning of November, Google finally released the version 1.0.0 of the Architecture Components, which is a Collection of Libraries for Android that establishes some foundations to help to build a good App architecture, such as Lifecycles, LiveData, ViewModel, Room, and Paging.
These libraries shows us a responsible effort from Google to present these Architecture Components, that provides a good foundation for the architecture of an App, as a way to simplify the work needed to build some of these good practices intrinsic to each of these libraries.
Sample
In order to add Room to your project, we need to modify build.gradle
dependencies {
…
compile
‘android.arch.persistence.room:runtime:1.0.0’
annotationProcessor
‘android.arch.persistence.room:compiler:1.0.0’
}
IIn order to setup a Database on Room, we need to have a Database that
exposes Daos to access data using Entities.
To help you to understand this way better, we will show you a small example of a Database with a single Table to store Persons.
Entity
Let’s start with Entities first: An Entity is a class with fields that
represent a Table by using Room’s Annotations to characterize any
non-regular field on the table, as the PrimaryKey, ForeignKey and
ColumnInfo.
@Entity
public
class Person {
@PrimaryKey(autoGenerate =
true)
public
int id;
public String name;
public Person(String
name) {
this.name = name;
}
}
Our example is a Table Person with an incremental Integer as id and a String as Name.
Dao
DAO ( Data Access Object) is an interface that declares methods to access data which requires Room’s Annotations to identify operations. We have Insert, Update and Delete operations that are usually validated based on the parameters.
For querying the data, we need to use Query annotation and also specify the SQL query that such methods will execute. These Queries use named parameters in order to accept parameters from the methods.
@Dao
public
interface PersonDao
{
@Insert
void
insertPerson(Person person);
@Update
int updatePerson(Person
person);
@Delete
int deletePerson(Person
person);
@Query(“select * from person where id = :id”)
Person getPerson(int
id);
@Query(“select count(*) from person”)
int
getTotalPersons();
}
Our PersonDao allows to insert, update and delete a Person. Allows, also, to get a Person by id and to get a count of the total of Persons.
Database
Room database specification is an abstract class that lists the entities accessed as the DAOs that we want to expose.
@android.arch.persistence.room.Database(entities = {Person.class}, version =
1)
public
abstract
class AppDatabase
extends RoomDatabase
{
public
abstract PersonDao
personDao();
}
Our example of Database only includes the access to the Person table, and only requires to expose the personDao.
This is a very small example of a Database, but allow us to quickly understand that we don’t need to access any SQLiteOpenHelper, SQLiteDatabase, Cursor, or ContentValues in order to manage our database.
For more information, you can take a look at the Room’s codelab and learn some tips on how to migrate your app with 7 Steps To Room.
Related Posts
Subscribe for Authentic Insights & Updates
We're not here to fill your inbox with generic tech news. Our newsletter delivers genuine insights from our team, along with the latest company updates.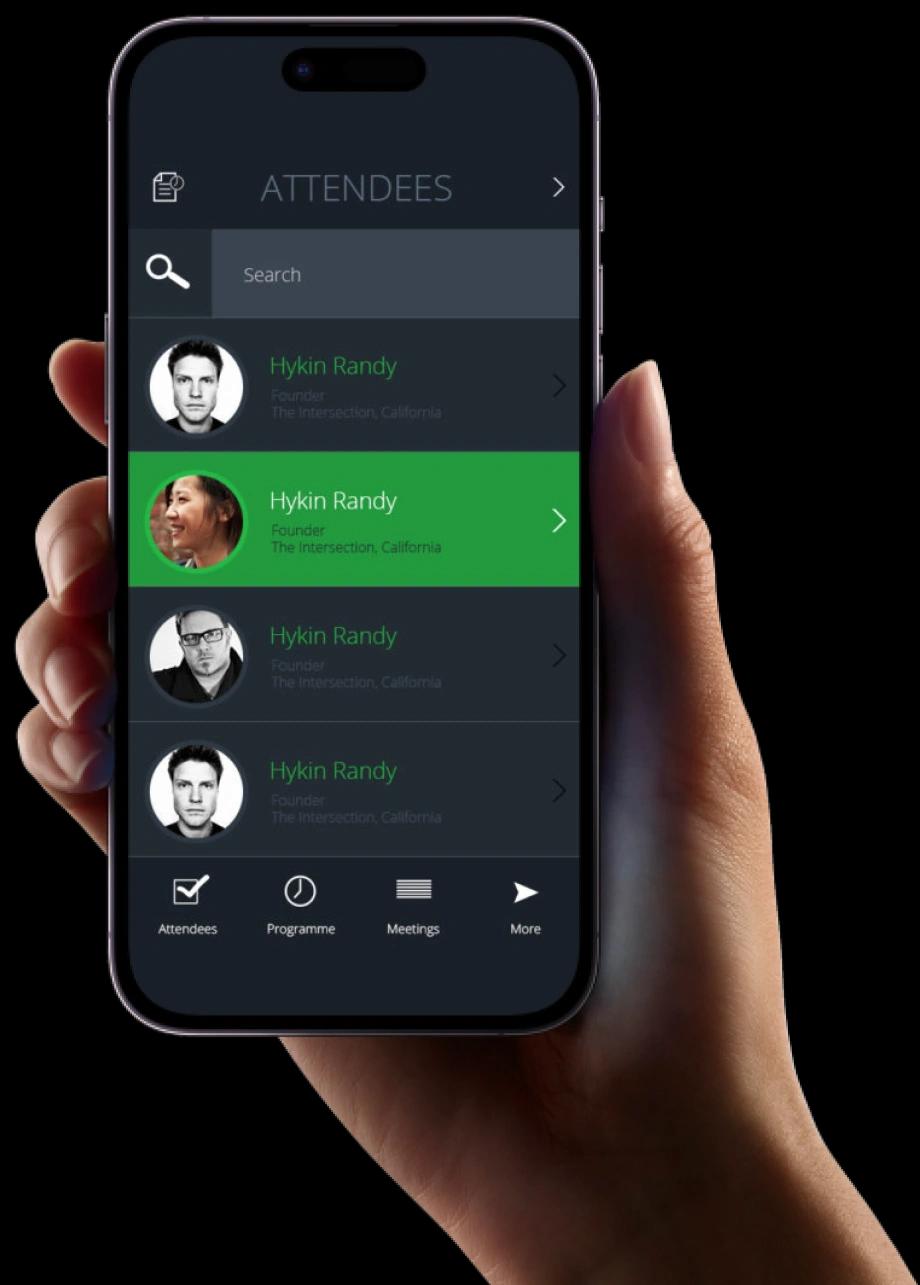