Exaud Blog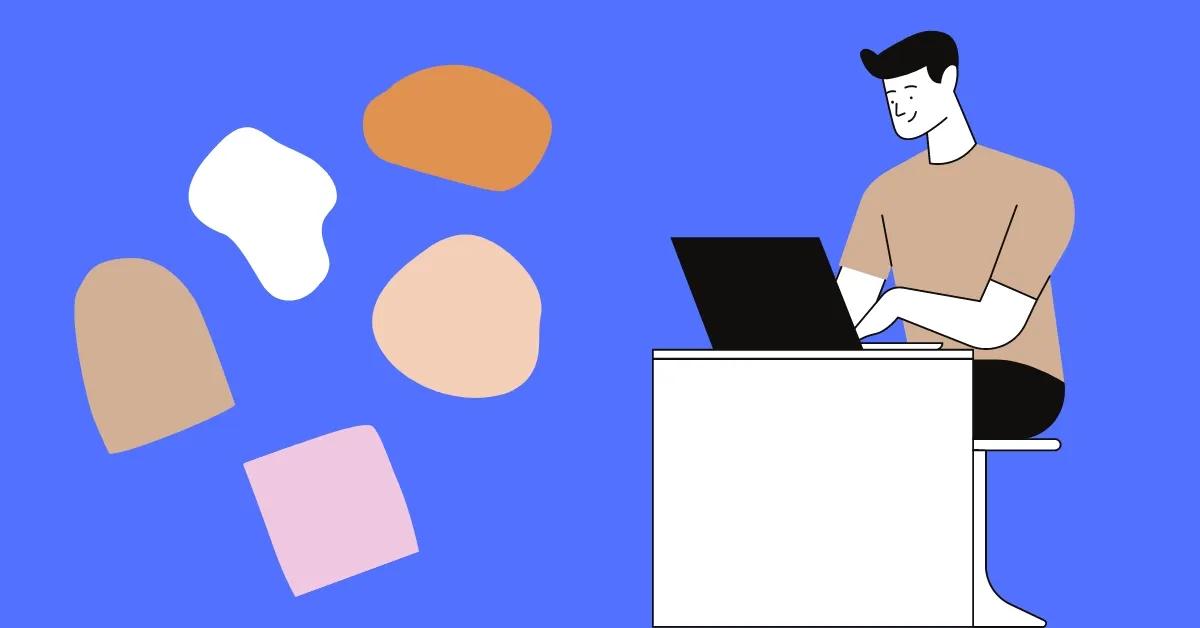
Blog
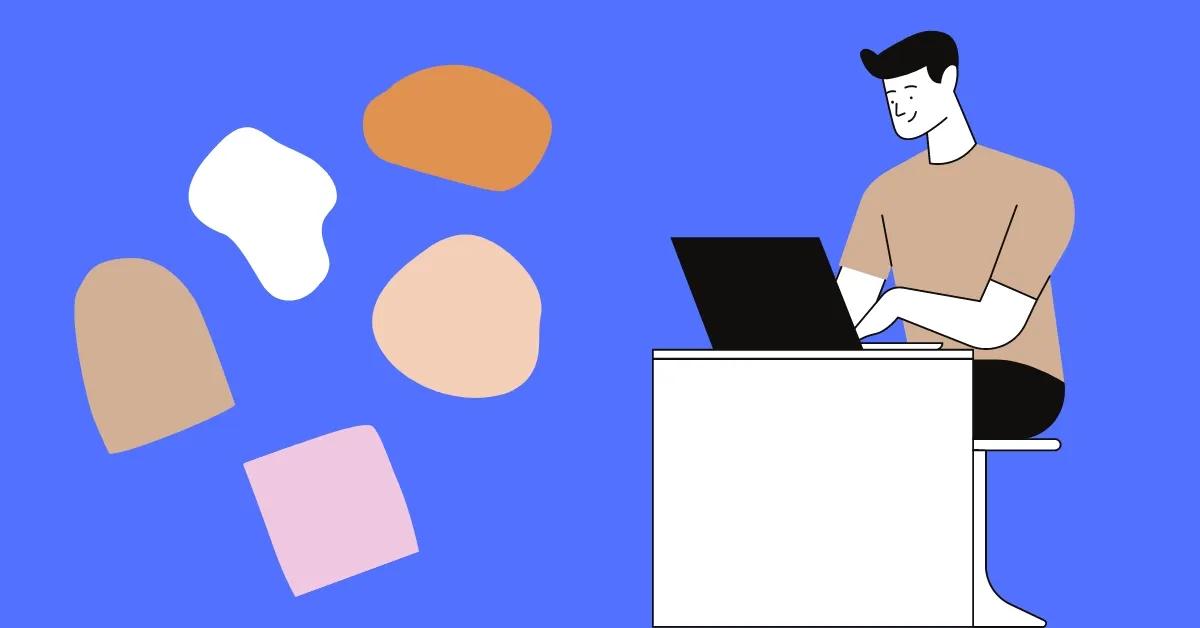
Detecting Shapes and Colors with Emgu CV
Our Senior Developer, Gil Moutinho, opens the magical door to an open-source tool capable of doing pretty awesome stuff. Check it out!Posted onby Gil MoutinhoImagining the Future of Technology
When I was younger, I liked to imagine what the future would be like at a technological level. I imagined cars levitating and moon bases, but instead, we have a global pandemic. Sigh… However, there are already some things that look like they came out of a science fiction movie. Countless computer programs recognize our faces; we can search for stuff on the internet using pictures of that same stuff, and there are even cars that can drive themselves. But still without turbo boost.
Introduction to OpenCV
Although the technologies I mentioned usually use their own ultra-advanced software, there is an open-source tool that can help us do these kinds of things. Do not expect to program an autonomous vehicle with it, but an application that can detect traffic signals and people through a camera is perfectly possible. I am obviously talking about OpenCV. An awesome computer vision library.
Emgu CV: The .NET Wrapper for OpenCV
Today, I am going to show you parts of a small application that I made using this technology, or better yet, using Emgu CV, which is an Open CV wrapper for .NET languages. This of course because C# is the best programming language in the world 😉.
Creating a Simple Application
This application is very simple. It detects circles and squares through the webcam, as well as colors, more specifically, red, green, and blue. Using a VideoCapture object and a timer, frames are sent to Emgu CV analyze, but first, these must be converted to grayscale:
using (Image<Bgr, byte> nextFrame = videoCapture.QueryFrame().ToImage<Bgr, byte>())
{
if (nextFrame != null)
{
Image<Gray, byte> grayframe = nextFrame.Convert<Gray, byte>();
…
}
}
Detecting Geometric Shapes
To detect circles, the Hough transform is used (I will not explain what this does in detail since I don’t have enough knowledge about it, but it’s basically an algorithm that detects geometric shapes in digital images):
CircleF[] circles = CvInvoke.HoughCircles(image, HoughType.Gradient, dp, minDist, cannyThreshold, circleAccumulatorThreshold, minRadius, maxRadius);
To detect rectangles, first, edges are found using the Canny edge detector (another tricky algorithm that discovers edges in an image):
CvInvoke.Canny(image, cannyEdges, cannyThreshold, cannyThresholdLinking);
Then, it is necessary to obtain the contours using the following method:
CvInvoke.FindContours(cannyEdges, contours, null, RetrType.List, ChainApproxMethod.ChainApproxSimple);
And for each contour found, try to generate polygons:
CvInvoke.ApproxPolyDP(contour, approxContour, CvInvoke.ArcLength(contour, true) * 0.05, true);
If the polygon has 4 vertices (approxContour.Size == 4), we are in the presence of a quadrilateral. But that does not mean that it is a rectangle. To be one, its internal angles must have 90°.
So, let us use the following algorithm to check if the quadrilateral at least looks like a rectangle:
Point[] pts = approxContour.ToArray();
LineSegment2D[] edges = PointCollection.PolyLine(pts, true);
for (int j = 0; j < edges.Length; j++)
{
double angle =
Math.Abs(edges[(j+1) % edges.Length].GetExteriorAngleDegree(edges[j]));
if (angle < 80 || angle > 100)
{
isRectangle = false;
break;
}
}
Color Detection
Finally, to detect colors, and since only additive primary colors (red, green, and blue) are being spotted, we just need to check if the color we’re looking for is between 150 and 255 of the RGB color model, and if the remaining primary colors are between 0 and 50, using the following method:
var image = rgbimage.InRange(new Bgr(0, 0, 150), new Bgr(50, 50, 255));
Using HSV for Non-Primary Colors
For non-primary colors, it would make sense to use the hue of the HSV model. Emgu CV finds the pixels with colors within the range that we defined, and then, we just need to have a threshold to decide the quantity of those colors that must appear in our camera to trigger the actions we want:
if (image.GetSum().Intensity > threshold)
Application Behavior
In the application that was developed, when a square is found, the geometric figure in the center of the screen grows, and when a circle is found, it rotates. When large quantities of one of the following colors are detected: red, green, or blue; the figure changes to that same color.
Exaud Services
At Exaud, we specialize in developing innovative software solutions that leverage advanced technologies like computer vision. Whether you need custom applications that utilize image recognition, machine learning, or data analysis, our team is ready to turn your ideas into reality. We are dedicated to providing top-notch development services that enhance your projects and drive efficiency.
Related Posts
Subscribe for Authentic Insights & Updates
We're not here to fill your inbox with generic tech news. Our newsletter delivers genuine insights from our team, along with the latest company updates.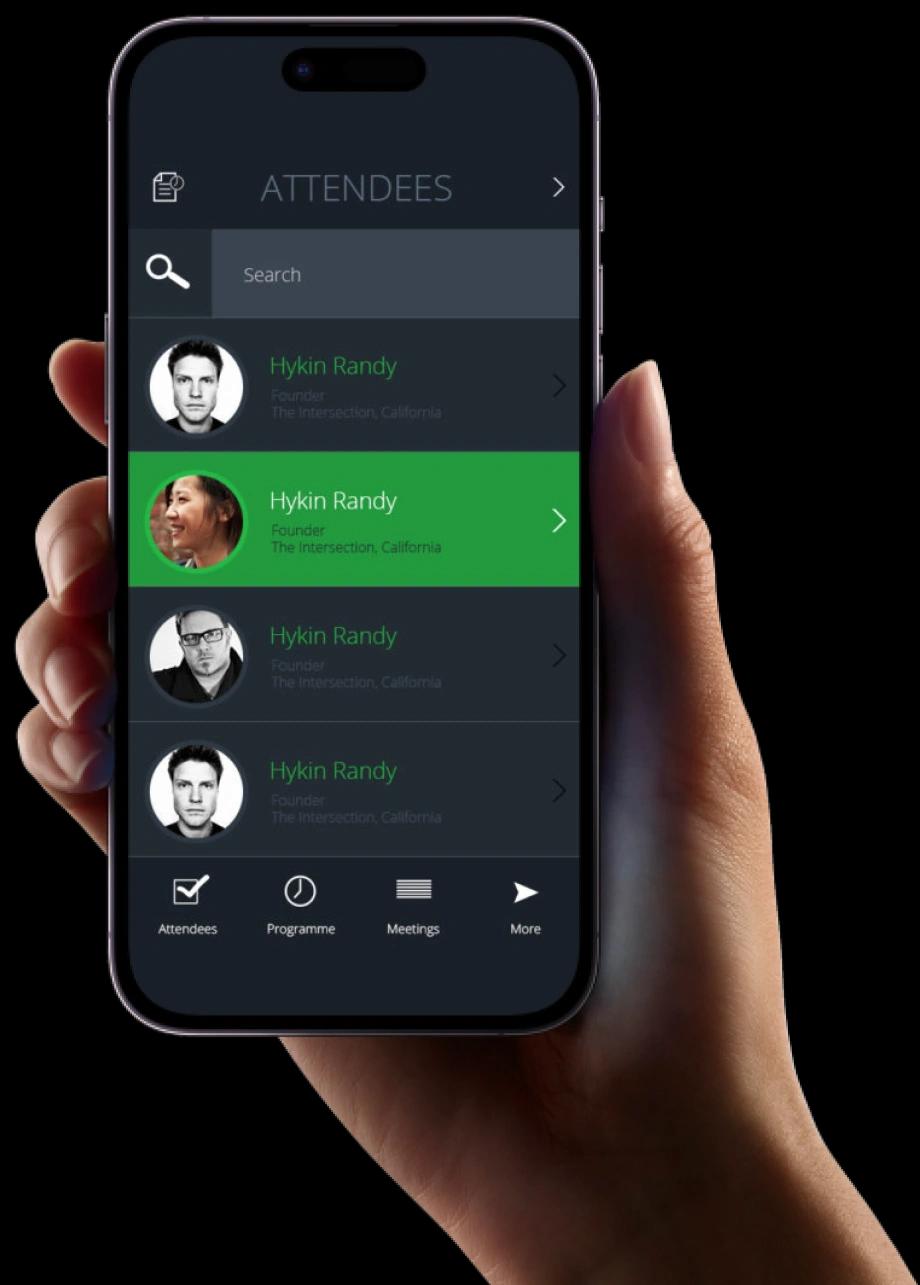